Want to create custom social sharing buttons for your WordPress website without installing a plugin? Then you are in the right place.
What happens when you install a plugin on your WordPress website? It decreases the performance of your website, and most of the plugins come with bloated codes, & scripts.
This article will explain how you can create custom social sharing buttons and implement them on your WordPress or any other websites.
So, let’s get started.
Create an HTML File
First, we need to create a custom social sharing button ‘index.html
‘ file, using any text editor (VS Code Recommended). However, the index.html file will not require in the end, cause WordPress already has one. We just created it for visualization, afterward, we will see how to implement it in WordPress.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>YOUR TITLE</title>
<link rel="stylesheet" href="style.css">
<!-- FontAwesome Icon CSS - Copy This -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.2/css/all.min.css"
integrity="sha512-HK5fgLBL+xu6dm/Ii3z4xhlSUyZgTT9tuc/hSrtw6uzJOvgRr2a9jyxxT1ely+B+xFAmJKVSTbpM/CuL7qxO8w=="
crossorigin="anonymous" />
<script src="/main.js">
</script>
</head>
<body>
<!-- Social Share Buttons HTML - Copy These -->
<div class="share-btn-container">
<a href="#" class="facebook-btn">
<i class="fab fa-facebook"></i>
</a>
<a href="#" class="twitter-btn">
<i class="fab fa-twitter"></i>
</a>
<a href="#" class="pinterest-btn">
<i class="fab fa-pinterest"></i>
</a>
<a href="#" class="linkedin-btn">
<i class="fab fa-linkedin"></i>
</a>
<a href="#" class="whatsapp-btn">
<i class="fab fa-whatsapp"></i>
</a>
<!-- You Can Put Here Other Social Media Icons-->
</div>
<!-- Dummy Content - No Need To Copy -->
<div class="content">
<h1>Floating Social Media Share Button HTML</h1>
<img src="https://dummyimage.com/768x403/000/fff.png&text=Floating+Social+Share+Button" alt="feature image"
class="feature">
<p>
Lorem ipsum dolor sit amet consectetur adipisicing elit. Reiciendis porro culpa quos facere non pariatur
doloremque dolorem quas, blanditiis doloribus aliquid tenetur eligendi itaque autem? Sapiente reiciendis
obcaecati alias quisquam.
Facere voluptate at pariatur, minima veniam nostrum vel sint velit praesentium modi culpa, dolore excepturi
necessitatibus debitis fuga quia quo recusandae dolores. Numquam, laudantium. Reiciendis, dolorem ipsa.
Recusandae, reprehenderit. Officia?
</p>
</div>
</body>
</html>
Create a CSS File
After creating the HTML file for the social sharing buttons, we need to create a ‘style.css
‘ file and copy and paste the into the CSS file as mentioned below. You can do customization if you want to change the looks of the social sharing buttons.
/* No Need Copy For Social Media Buttons*/
.content {
padding: 100px;
font-family: Georgia, 'Times New Roman', Times, serif;
font-size: large;
line-height: 1.5em;
}
.feature {
display: block;
margin-left: auto;
margin-right: auto;
width: 50%;
box-shadow: 5px 5px 0 darkgray;
}
/* No Need Copy For Social Media Buttons*/
/* Copy From Here - Floating Social Share Link */
.share-btn-container {
background: rgba(0, 0, 0, 0);
display: inline-flex;
flex-direction: column;
padding: 15px;
box-shadow: 0 4px 8px rgb(0 0 0 / 30%);
position: fixed;
top: 50%;
transform: translateY(-50%);
border-radius: 0 15px 15px 0;
}
.share-btn-container a i {
font-size: 32px;
}
.share-btn-container a {
margin: 12px 0;
transition: 500ms;
}
.share-btn-container a:hover {
transform: scale(1.2);
}
.share-btn-container .fa-facebook {
color: #3b5998;
}
.share-btn-container .fa-twitter {
color: #1da1f2;
}
.share-btn-container .fa-linkedin {
color: #0077b5;
}
.share-btn-container .fa-pinterest {
color: #bd081c;
}
.share-btn-container .fa-whatsapp {
color: #25d366;
}
/* Hide Social Share On Mobile */
@media (max-width: 768px) {
.share-btn-container {
display: none;
}
}
/* Floating Social Share Link */
Create a JavaScript File
After creating the CSS file and HTML file for the custom social sharing buttons, we need to create a JavaScript ‘main.js
‘ file and copy the code from below and paste it into the JavaScript file.
const ffbBtn = document.querySelector(".facebook-btn");
const ftwBtn = document.querySelector(".twitter-btn");
const fpiBtn = document.querySelector(".pinterest-btn");
const fliBtn = document.querySelector(".linkedin-btn");
const fpoBtn = document.querySelector(".pocket-btn")
const fwaBtn = document.querySelector(".whatsapp-btn")
function init() {
let postUrl = encodeURI(document.location.href);
let postTitle = encodeURI("Hi everyone, please check this out: ");
let via = encodeURI("itsmepronay");
ffbBtn.setAttribute(
"href",
`https://www.facebook.com/sharer.php?u=${postUrl}`
);
ftwBtn.setAttribute(
"href",
`https://twitter.com/share?url=${postUrl}&text=${postTitle}&via=${via}`
);
fpiBtn.setAttribute(
"href",
`https://pinterest.com/pin/create/bookmarklet/?url=${postUrl}&description=${postTitle}`
);
fliBtn.setAttribute(
"href",
`https://www.linkedin.com/shareArticle?url=${postUrl}&title=${postTitle}`
);
fpoBtn.setAttribute(
"href",
`https://getpocket.com/save?url=${postUrl}&title=${postTitle}`
);
fwaBtn.setAttribute(
"href",
`https://wa.me/?text=${postTitle} ${postUrl}`
);
}
init();
Run Local Files On Browser
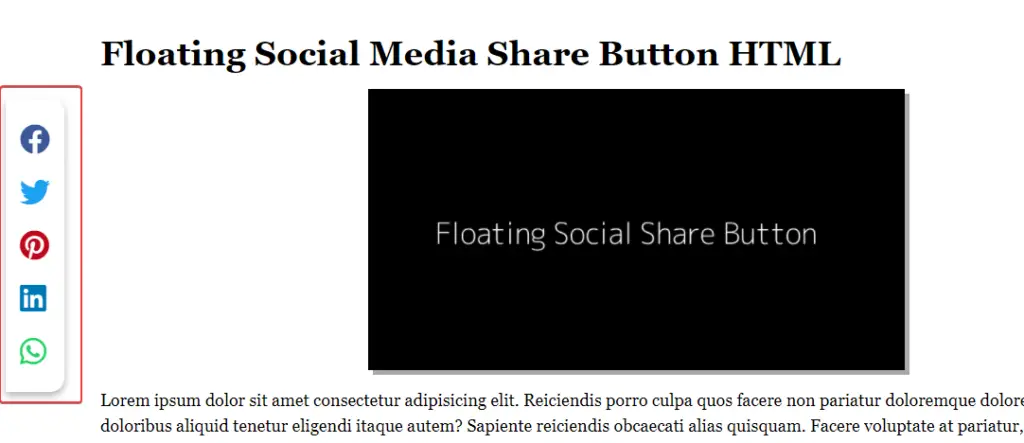
In VS code, you can install an extension name “Live Server”, using that you can check your codes in a browser with live changes. As we have created all these files for social share buttons, and when we saw it in live server mode, it worked perfectly.
Read More About WordPress Customization:
Implement Custom Social Sharing Buttons On WordPress
In some instances, implementing custom social sharing buttons would be easy, like if you are using a premium theme like GeneratePress Premium, Astra Pro, or Neve Pro. These themes have custom hooks feature, so if you use one of the themes, just hook the code.
If you are using a free WordPress theme, then code implementation could be hard for you, but I will show all possible ways to implement social sharing buttons in this article.
We have used the GeneratePress Premium theme for tutorials, and you can implement code in other premium themes such as Astra Pro, Neve Pro, and Kadence Pro themes using the same method.
Step – 1. Add Custom Hook
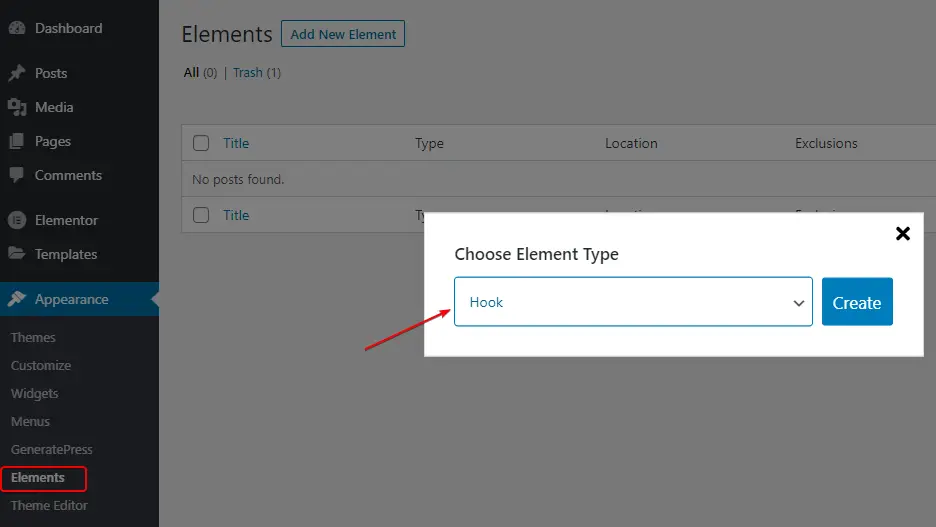
Step – 2. Insert HTML & Js Code
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.2/css/all.min.css" integrity="sha512-HK5fgLBL+xu6dm/Ii3z4xhlSUyZgTT9tuc/hSrtw6uzJOvgRr2a9jyxxT1ely+B+xFAmJKVSTbpM/CuL7qxO8w==" crossorigin="anonymous" />
<div class="share-btn-container">
<a href="#" target="_blank" class="facebook-btn">
<i class="fab fa-facebook"></i>
</a>
<a href="#" target="_blank" class="twitter-btn">
<i class="fab fa-twitter"></i>
</a>
<a href="#" target="_blank" class="pinterest-btn">
<i class="fab fa-pinterest"></i>
</a>
<a href="#" target="_blank" class="linkedin-btn">
<i class="fab fa-linkedin"></i>
</a>
<a href="#" target="_blank" class="whatsapp-btn" style="color: #ea4c89;">
<i class="fab fa-whatsapp"></i>
</a>
<a href="#" target="_blank" class="pocket-btn">
<i class="fab fa-get-pocket" style="color:#ee4056;"></i>
</a>
</div>
<script>
const ffbBtn = document.querySelector(".facebook-btn");
const ftwBtn = document.querySelector(".twitter-btn");
const fpiBtn = document.querySelector(".pinterest-btn");
const fliBtn = document.querySelector(".linkedin-btn");
const fpoBtn = document.querySelector(".pocket-btn")
const fwaBtn = document.querySelector(".whatsapp-btn")
function init() {
let postUrl = encodeURI(document.location.href);
let postTitle = encodeURI("Hi everyone, please check this out: ");
let via = encodeURI("itsmepronay");
ffbBtn.setAttribute(
"href",
`https://www.facebook.com/sharer.php?u=${postUrl}`
);
ftwBtn.setAttribute(
"href",
`https://twitter.com/share?url=${postUrl}&text=${postTitle}&via=${via}`
);
fpiBtn.setAttribute(
"href",
`https://pinterest.com/pin/create/bookmarklet/?url=${postUrl}&description=${postTitle}`
);
fliBtn.setAttribute(
"href",
`https://www.linkedin.com/shareArticle?url=${postUrl}&title=${postTitle}`
);
fpoBtn.setAttribute(
"href",
`https://getpocket.com/save?url=${postUrl}&title=${postTitle}`
);
fwaBtn.setAttribute(
"href",
`https://wa.me/?text=${postTitle} ${postUrl}`
);
}
init();
</script>
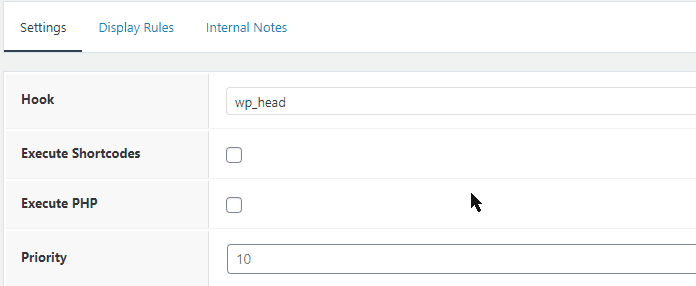
Step – 3. Add Custom CSS
...
/* Copy From Here - Floating Social Share Link */
.share-btn-container {
background: rgba(0, 0, 0, 0);
display: inline-flex;
flex-direction: column;
padding: 15px;
box-shadow: 0 4px 8px rgb(0 0 0 / 30%);
position: fixed;
top: 50%;
transform: translateY(-50%);
border-radius: 0 15px 15px 0;
}
.share-btn-container .fa-facebook {
color: #3b5998;
}
...
/* Copy Full Code From Above */
Implement Custom Social Media Sharing Buttons For Free Theme Users
If you are using a free WordPress theme and want to implement the custom social sharing button, then open the Theme editor and paste the HTML & JS code as shown in the image below.
Step – 1. Add Custom HTML & JS Code
By copying the HTML and JavaScript code from above, you have to paste it into the ‘header.php‘ file.
You have to open Appearance, then Theme editor, and then select header.php.
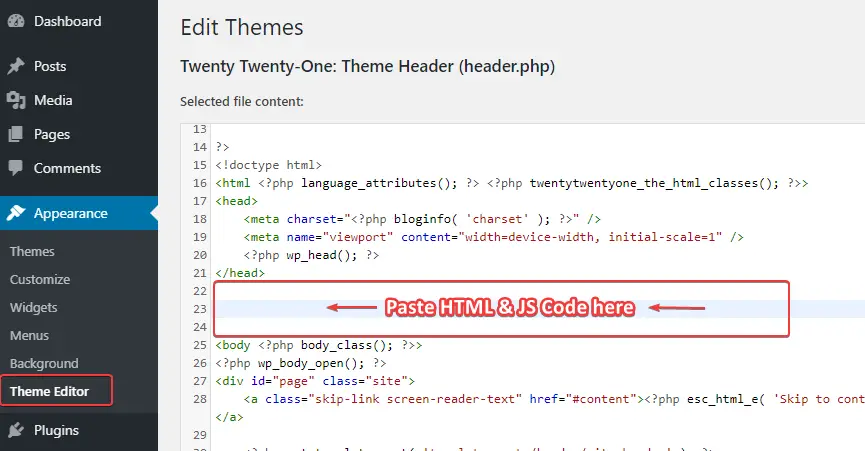
Step – 2. Add Custom CSS
After adding the custom social sharing buttons HTML and JavaScript code, you must add custom CSS. To add CSS, you have to open Appearance and then ‘Customize.’
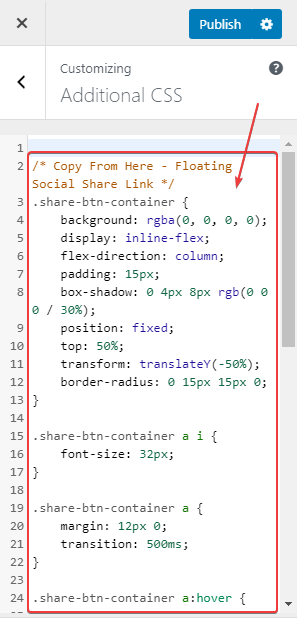
FAQs About Custom Social Sharing Buttons
How to add the WhatsApp share button in HTML?
You can use the social warfare plugin to add WhatsApp social share buttons on your website, and otherwise, you can use the code of the custom social sharing buttons above.
How do I add social media buttons to my website?
To add custom social sharing buttons to your website, you can use the above custom HTML, CSS, and JavaScript on your website. We have also explained how to implement it.
How do I add social buttons in WordPress?
You can use plugins on the WordPress platform such as Social Snap and Social Warfare to add social sharing buttons to posts.
Conclusion
If you want to create a custom social sharing button for your website, use the above tutorial.
If you use a plugin for social sharing buttons on your WordPress, your website’s performance can be reduced.
So if you want your website’s performance is not low, you can use custom social sharing buttons on your WordPress and custom website.
If you have any problems with adding custom social sharing buttons, you can tell us in the comment section.